Webhook Documentation
Introduction
TransactAPI has webhooks which help you get notifications when TransactAPI methods respond with success. By using these webhooks, this alleviates the need to request a "get" method continuously to check on a status.
What is an webhook?
A webhook (also called a web callback or HTTP push API) is a way to exchange real-time information and updates automatically within two systems. Webhooks receive calls through HTTP POST requests only when the connected external system has a data update. APIs will place calls for data whether there's been a data update response, or not. This makes webhooks much more efficient for both provider and consumer.
While webhooks are an effective tool, they require an Internet connection between the data source and the web server to function. Additionally, a script must be present on the server at the destination URL and it must be able to recognize and parse the POST data. If the Internet connection or script is not available, the webhook will not work.
Creating webhooks and notifications
To create a webhook:
- Navigate to the TransactAPI admin panel. On the Dashboard pane find the "View Webhooks" quick link. it it located under the "Administrative" section.
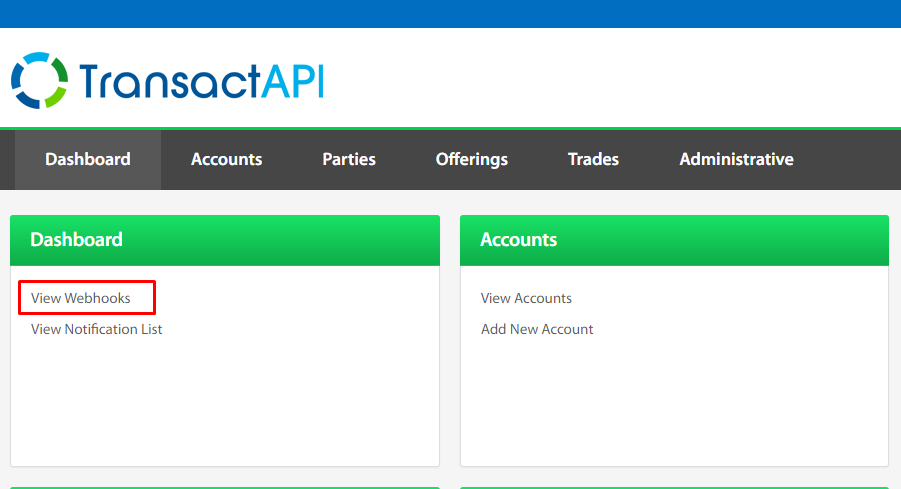
- On the webhook page select the "Add Webhook" button in the top left corner. In the popup box select the webhook trigger you want. For example if you want you webhook to run when an account is created choose "createAccount". Then add your URL, this should be a URL that you have setup to listen for a POST method. If you have not used webhooks before we recommend using ngrok to setup a temporary URL for testing.
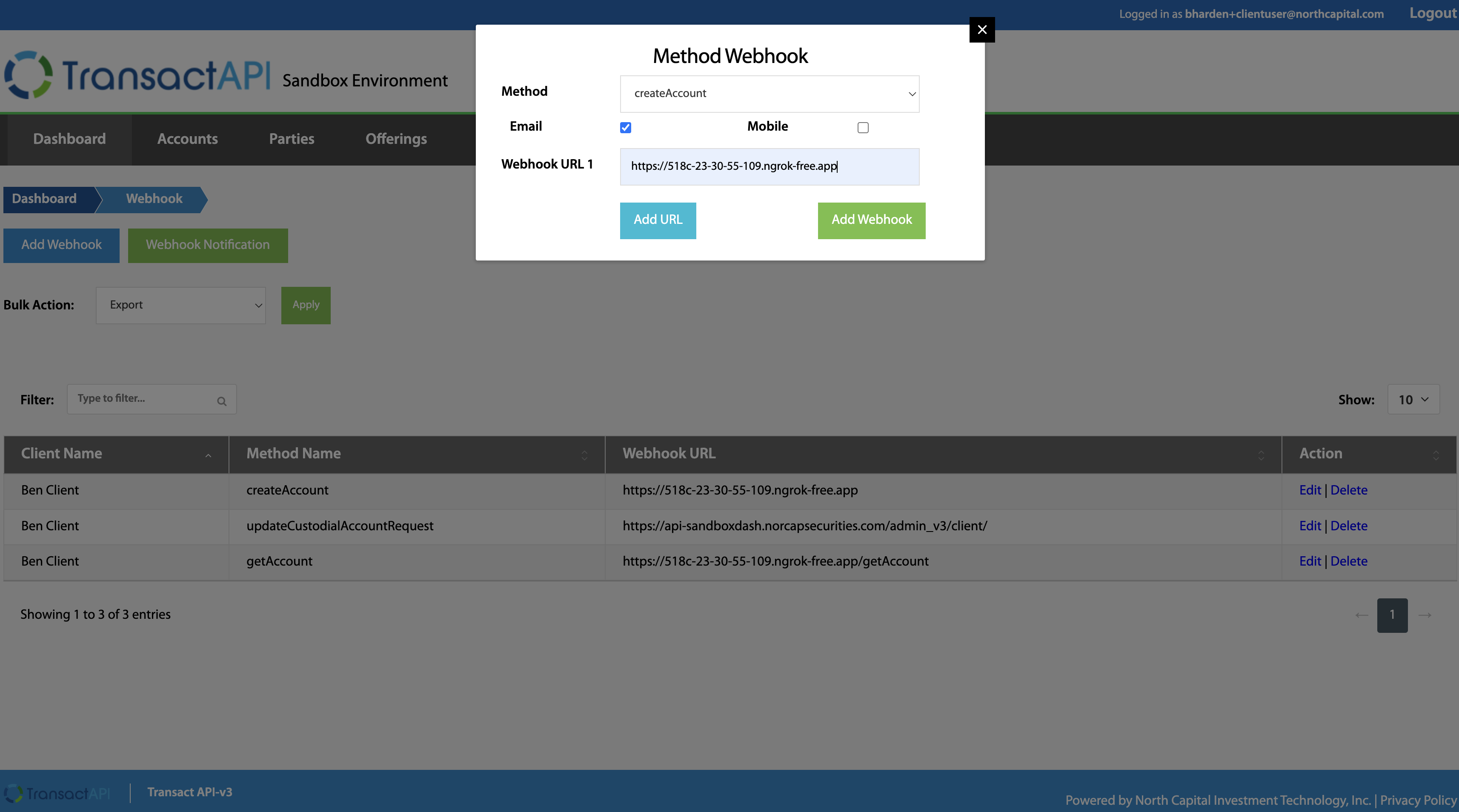
- Your code should be listening for POST requests. Here is an example using ngrok and Node.js.
Check that node.js and npm are installed on your machine
node -v
npm -v
Create a new folder called webhooks. Then create a new file called webhooks.js
mkdir webhooks
touch webhook.js
Add this node.js code to you webhooks/webhook.js file
const express = require("express");
const bodyParser = require("body-parser");
const app = express();
const port = 3000; // Replace this with the port you want to use
// Middleware to parse incoming request data as JSON
app.use(bodyParser.urlencoded());
// Route to handle the 'getAccount' webhook method
app.post("/", (req, res) => {
// Process the incoming webhook data for 'createAccount' method here
// The data sent by TransactAPI will be available in req.body
// You can access specific properties like req.body.accountId, req.body.accountType, etc.
// For simplicity, we'll just log the received data
console.log('Received "createAccount" webhook data:', req.body);
// Respond to the webhook request
res.status(200).send('Webhook for "getAccount" received successfully');
});
// Start the server
app.listen(port, () => {
console.log(`Webhook server is running on port ${port}`);
});
Install Express and Body Parser
npm install express body-parser
Start you node server
node webhook.js
You should see a message indicating that the server is running, for example: Webhook server is running on port 3000
Now navigate to https://ngrok.com/ and signup for a free account
Copy your authtoken then create a new folder called ngrok and set your auth token.
After signing up, download the ngrok executable for your operating system (Windows, macOS, or Linux)
Create a new folder called ngrok
Put the downloaded executable file in the ngrok folder
In the ngrok folder authenticate you account
//create folder ngrok
mkdir ngrok
//authenticate your ngrok account
cd ngrok
./ngrok authtoken YOUR_AUTH_TOKEN
Expose your local server to the public internet
./ngrok http PORT_NUMBER
After running the ngrok http command, ngrok will generate a temporary public URL, which you can use to access your local server from the internet.
Look for the "Forwarding" section in the ngrok terminal output. You will see a URL in the format http://xxxxxx.ngrok-free.app. This URL is your temporary public address
Now you can navigate to the TransactAPI admin website and add your new URL "http://xxxxxx.ngrok-free.app" as the "Webhook URL 1" in the popup that appears when hit "AddWebhook". Don't forget to set you trigger method to "createAccount"
Now use the method of your choice (Postman, Custom Code, Admin Panel, etc...) to call the createAccount API endpoint. When that endpoint completes you will see your logs in the Node.js logs that we setup earlier.
Some sample PHP code
if ($conn->connect_error)
{
die("Connection failed: " . $conn->connect_error);
}
if (isset($_POST['partyId']))
{
$partyId = $_POST['partyId'];
$offeringId = $_POST['offeringId'];
$orderStatus = $_POST['orderStatus'];
$insert = mysqli_query($conn, "INSERT INTO checkworld (partyId,offeringId,orderStatus) VALUES ('$partyId','$offeringId','$orderStatus')")
or die(mysqli_error($conn));
print_r($insert);
}
You can also make these triggers send you emails or text messages
Just find the "View Notifications List" quick link on the Dashboard Pane and navigate to the Notifications page. Then click the "Add Notification" Button in the top left corner and add and email and phone number you would like to receive notifications at.
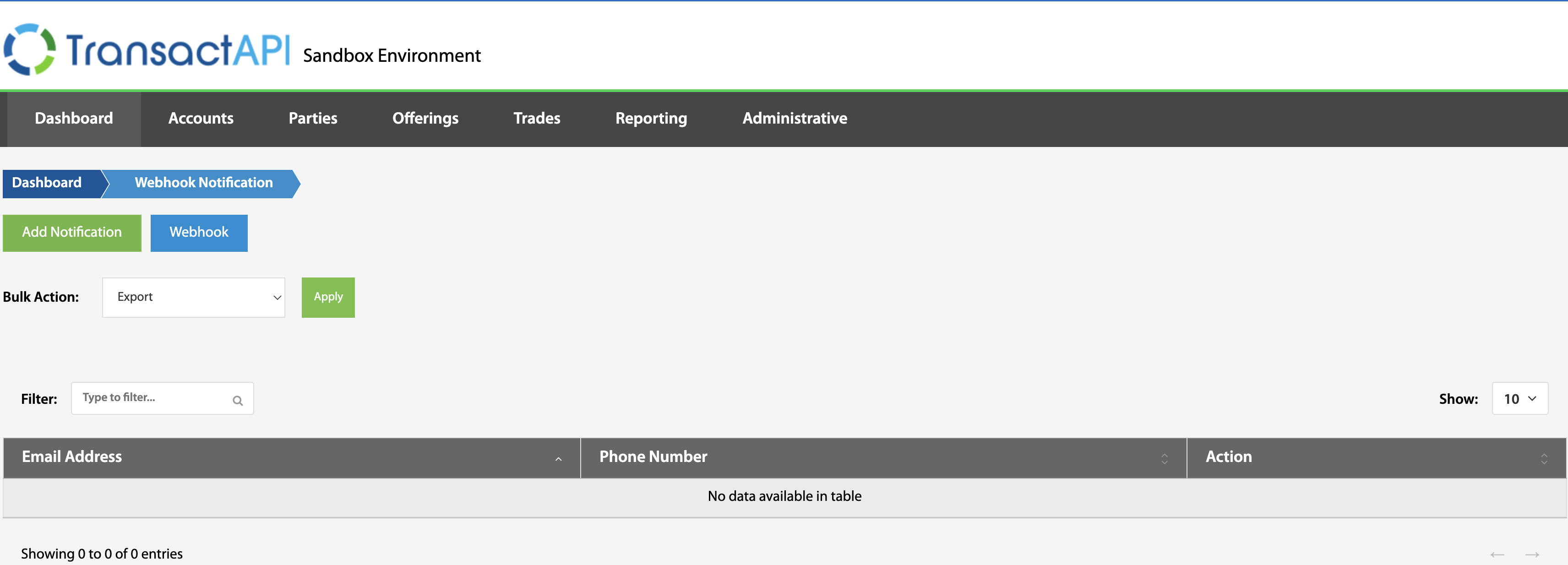
Webhook Method List
Below is the complete list of webhook methods available in the TransactAPI. Examples are shown in JSON format. Webhooks may require parsing.
Method | Description | Example |
---|---|---|
cancelInvestment | The webhook is triggered when cancelInvestment is invoked, either via API or via the TransactAPI dashboard. | {"accountId":"AXXXXXX","OrderId":"4795XXX","Transaction Type":"ACH","OrderStatus":"CANCELED"} |
cancelOffering | This webhook is triggered when an offering is cancelled via "cancelOffering" method. | |
ccFundMove | This webhook is triggered when an online credit card transaction is performed. | {"tradeId":"8916000","ccreferencenumber":"682XXXXXX"} |
ccFundMovement | This webhook is triggered when an online credit card transaction is performed. | {"accountId":"A59XXXXXX","tradeId":"981XXXXXX","offeringId":"74XXXXXX","totalAmount":"10.000000","ccreferencenumber":"138XXXXXX","fundStatus":"Pending","transactionstatus":"Pending"} |
closeOffering | This webhook is triggered when an offering is closed via the "closeOffering" method. | {"offeringId":"60XXXX","offeringStatus":"CLOSED"} |
createAccount | This webhook is triggered when a new account is created. | {"accountId":"A25XXXXXX","kycStatus":"PENDING","amlStatus":"PENDING","accreditedStatus":"NOT_ACCREDITED","approvalStatus":"APPROVED"} |
createExternalAccount | The webhook is triggered when a new external account is created. | {"accountId":"A92XXXXXX","ExtAccountfullname":"Test Ext Account","Extnickname":"Checking TEST","ExtRoutingnumber":"MDExMDAxNzI2","ExtAccountnumber":"MDAxMTgyMjc4MA==","types":"Account","accountType":"Checking"} |
createLink | This webhook is triggered when a link is created. | {"id":"53XXXX","firstEntryType":"Account","firstEntry":"A68XXXXXX","relatedEntry":"P27XXXXXX","relatedEntryType":"IndivACParty","linkType":"member","notes":"Notes"} |
createMaaSUser | This webhook is triggered when a new user is created in the Marketplace-as-a-Service (MaaS) platform. | |
createOrder | This webhook is triggered when a new order is created on the PPEX/ATS. | {"id":"66XXXX","securityId":"8XXXX","orderId":"350XXXXXX","action":"Bid","orderStatus":"Live","token":"123456"} |
createParty | This webhook is triggered when a new party is created. | {"partyId":"P64XXXXXX","KYCstatus":null,"AMLstatus":null} |
createTrade | This webhook is triggered when a new trade is created in an offering. | {"tradeId":"66XXXX","transactionId":"21XXXXX","transactionAmount":"100.000000","transactionDate":"2023-06-29 13:49:07","transactionStatus":"CREATED","RRApprovalStatus":"PENDING","RRName":"N/A","RRApprovalDate":null,"PrincipalApprovalStatus":"PENDING","PrincipalName":"N/A","PrincipalDate":null,"closeId":null,"eligibleToClose":"no"} |
deleteExternalAccount | This webhook is triggered when a linked bank account has been deleted from the associated account. | {"accountId":"A54XXXXXX","Ext_status":"Deleted"} |
deleteLink | This webhook is triggered when a link is deleted. | |
deleteMaaSUser | This webhook is triggered when a MaaS user is deleted. | |
deleteOffering | This webhook is triggered when an offering has been deleted via the deleteOffering method. | |
deleteTrade | This webhook is triggered when a previously created trade is deleted (trade status is updated to “CANCELED”). | {"tradeId":"899XXXXXX"} |
editTrade | This webhook is triggered when trade information has been edited via the editTrade method. | {"tradeId":"713XXXXXX","shares":"30.000000","sharePrice":300,"totalShares":"1000.000000","remainingShares":"840.000000","closeId":null} |
editTradeUnits | This webhook is triggered when the unit amount or unit price is updated. | {"tradeId":"169XXXXXX","sharePrice":1500,"unitPrice":"10.00","totalShares":"150"} |
externalFundMove | This webhook is triggered when the fund movement has been initiated to move funds from the investors external account to the NCPS escrow account. | {"tradeId":"72XXXXX","RefNum":"153XXXXXX"} |
getAccount | This webhook is triggered when the getAccount API is called. | |
getCustodyFundsDisbursement | This webhook is triggered when the custody fund disbursement status is updated. | "accountId": "A98779676", "amount": "100", "type": "ACH", "routingSwiftId": "123467894", "accountIban": "15895555555555555", "additionalDetails": "notes", status": "Pending", "requestId": "0dBczf" |
linkExternalAccount | This webhook is triggered when an investor links their external account using Plaid. | {"clientId":"swXXXXxxxXXxX","params":"{"clientID":"swXXXXxxxXXxX","developerAPIKey":"xxXXxxXXxXXxxXXXXx","accountId":"A76XXXXXX"}","method":"linkExternalAccount","webhookurl":"<https://test-api2.pre-prod.testlabs.com/north-capital-proxy/webhooks/publish/c894fd53ff3/linkExternalAccount","webhookdata":"{"accountId":"A76XXXXXX","refNum":"983XXXXXX","account_number":"YVltc5tGr54f","routing_number":"xxxxxxxxxx","account_name":"EveryDay> Checking","account_type":"Checking","institutionId":"ins_15","institution_name":"Navy Federal Credit Union"}","methodtype":"POST","createdDate":"2023-06-29 14:33:26","createdIpAddress":"xxx.xx.xxx"} |
matchedTradenotify | This webhook is triggered when a trade is matched. | {"executionTime":"2022-09-19 16:29:12","numberOfShares":"2.000000","Price":"7.390000","askOrderId":"131XXXXXX","bidOrderId":"343XXXXXX","matchId":"148XXXXXX"} |
notifySettlement | This webhook is triggered when notifySettlement API is used to update a trades settlement status. | {"memberid":"A8XXXX","issuerid":"56XXXX","tradeID":"546XXXXXX","tradeStatus":"Canceled"} |
performKycAml | This webhook is triggered when KYC/AML enhanced is performed. | {"partyId":"P47XXXXXX","kycstatus":"Disapproved","amlstatus":"Disapproved"} |
performKycAmlBasic | This webhook is triggered when KYC/AML basic is performed. | {"partyId":"P71XXXXXX","kycstatus":"Disapproved","amlstatus":"Disapproved"} |
requestAiVerification | This webhook is triggered when Accredited Investor Verification is requested for an Investor’s account in TransactAPI. | {"accountId":"A08XXXXXX","aiRequestStatus":"Pending","airequestId":"z6Cu8r","accreditedStatus":"Pending"} |
requestCustodialAccount | This webhook is triggered when a Custodial Account is requested through the API. | |
requestForVoidACH | This webhook is triggered when the requestForVoidACH is used to update a pending transaction to void. | |
requestForVoidCCTransaction | This webhook is triggered when the requestForVoidCCTransaction is used to update a pending transaction to void. | {"accountId":"A49XXXXXX","tradeId":"709XXXXXX","transactionstatus":"Pending","fundStatus":"Voided","RefNum":"598XXXXXX","errors":""} |
updateAccount | This webhook is triggered when an account is updated. | {"accountId":"A49XXXXXX","suitabilityScore":"0","approvalStatus":"Pending"} |
updateAccountArchivestatus | This webhook is triggered when an account is archived. | {"accountId":"A03XXXXXX","archiveStatus":"1"} |
updateAiRequest | This webhook is triggered when new information has been uploaded by the investor and is ready for review, or if an updated letter is being requested. | {"accountId":"A7XXXXX","aiRequestStatus":"New Info Added","airequestId":"3MJUcU","accreditedStatus":"Verified Accredited"} |
updateAiVerification | This webhook is triggered when the Accreditation Verification status has been updated. (Ex: From ‘ Pending’ to ‘Need More Info’) | {"accountId":"A32XXXXXX","aiRequestStatus":"Approved","airequestId":"cxXcDv","accreditedStatus":"Verified Accredited"} |
updateCCFundMoveStatus | This webhook is triggered when the Transaction Status for CreditCard is updated. (Ex: From ‘Pending’ to Submitted’) | {"accountId":"A49XXXXXX","tradeId":"20XXXXXX","transactionstatus":"Approved","fundStatus":"Settled","RefNum":"9621XXXXX","errors":"","vantivTransactionMessage":"Approved"} |
updateCustodialAccountRequest | This webhook is triggered when new information has been added to an existing custodial account request. | |
updateDocuSignStatus | This webhook is triggered when the status of a DocuSign is updated. (Ex: From Out for Signature to Completed) | {"id":"12XXXX","documentId":"2410b924-cfd7-4409-XXXX-e7808x7xhXX","esignstatus":"SIGNED","updatedDate":"2023-01-25 12:30:27","updatedIpAddress":"111.11.1.111","tradeId":"38XXXXXX"} |
updateEligibleToCloseStatus | This webhook is triggered when the Escrow status has been updated to 'Eligible to Close'. | {"tradeId":"740XXXXXX","eligibleToClose":"Yes"} |
updateEntity | This webhook is triggered when an entity party is updated. | {"partyId":"E3XXXXX","KYCstatus":null,"AMLstatus":null} |
updateExternalAccount | This webhook is triggered when an external bank account is updated. | {"accountId":"A98XXXXX","ExtAccountfullname":"Test Name","Extnickname":"High Yield Savings Acct - American Express - Personal Savings","ExtRoutingnumber":"MS8jske8JF","ExtAccountnumber":"MzIwMDA2UDY4NDAz","types":"Account","accountType":"Savings"} |
updateExternalFundMoveStatus | This webhook is triggered when the Transaction Status for ACH is updated. (Ex: From ‘Pending’ to Submitted’) | {"accountId":"A81XXXXXX","tradeId":"315XXXXXX","transactionstatus":"Approved","fundStatus":"Settled","RefNum":"579XXXXXX","errors":""} |
updateKycAml | This webhook is triggered when the KYC/AML questions have been answered and the "updateKycAml" method has been invoked. | |
updateKycAmlRequest | This webhook is triggered when North Capital updates the KYC/AML status to "Needs More Info" during a manual review (only used in-conjunction with requestKycAml). | {"partyId":"P1XXXXXX","KYCstatus":"Needs More Info","AMLstatus":"Pending"} |
updateKycAmlStatus | This webhook is triggered when the "Update KYC/AML Status" button in the TransactAPI dashboard is utilized, which updates the status to "More Info Added" and notifies the North Capital verification team that KYC/AML is ready to re-review (only used in-conjunction with requestKycAml). | {"partyId":"P07XXXXXX","KYCstatus":"New Info Added","AMLstatus":"Auto Approved"} |
updateLinkExternalAccount | This webhook is triggered when an external account is updated using Plaid. | {"clientId":"swMOJLevn4KcZAP","params":"{"clientID":"swMOJLevn4KcZAP","developerAPIKey":"0revbTe3sjfX1SWo2JRjJKDCNpAyW5p2PRn","accountId":"A92643015"}","method":"updateLinkExternalAccount","webhookurl":"<https://test-api2.pre-prod.test.com/north-capital-proxy/webhooks/publish/c894fd53ff3/updateLinkExternalAccount","webhookdata":"{"accountId":"A9XXXXXXX","refNum":"64XXXXXXX","account_number":"bS9uWGdJNFJqY3ffwLzZISSDfzc0xvSF09","routing_number":"yQituaksdhfASYASDFpMbmk4Zz09","account_name":"******8444","account_type":"Checking","institutionId":"ins_11XXXX","institution_name":"Wealth Test"}","methodtype":"POST","createdDate":"2023-06-23> 22:01:31","createdIpAddress":"171.11.1.11"} |
updateMaaSUser | This webhook is triggered when updates to the Marketplace-as-a-Service user have been updated. | |
updateOffering | This webhook is triggered when an offering is updated in TransactAPI. | {"issuerId":"19XXX","offeringId":"10XXXXXX","developerAPIKey":"key here","issueName":"1755 Test","issueType":"Fund","targetAmount":"4514621.000000","minAmount":"500.000000","maxAmount":"4514621.000000","unitPrice":"5.000000","totalShares":"902924.200000","remainingShares":"814473.200000","startDate":"12-10-2022","endDate":"04-01-2024","offeringStatus":"Pending","offeringText":"Your offering text here.</p>","stampingText":"collab","escrowAccountNumber":"","field1":"","field2":"","field3":"","createdDate":"2022-08-31 02:30:57","createdIPAddress":null} |
updateOrder | ||
updateParty | This webhook is triggered when a party is updated. | {"partyId":"P13XXXXXX","KYCstatus":"Disapproved","AMLstatus":"Auto Approved"} |
updatePartyEntityArchivestatus | This webhook is triggered when a party is archived. | {"partyId":"P29XXXXXX","archiveStatus":"1"} |
updateTrade | This webhook is triggered when a trade is updated. | |
updateTradeArchivestatus | This webhook is triggered when the archive status of a trade is updated. | {"partyId":"P36XXXXXX","offeringId":"74XXXXXX","orderStatus":"CREATED","archiveStatus":"0","tradeId":"607XXXXXX"} |
updateTradeDocArchivestatus | This webhook is triggered when a document for a trade is archived. | |
updateTradeStatus | This webhook is triggered when the trade status has been updated. | {"id":"213XXXX","offeringId":"748XXXXX","accountId":"A49XXXXXX","partyId":"P59XXXXXX","party_type":"IndivACParty","escrowId":null,"transactionType":"CREDITCARD","totalAmount":"1.000000","totalShares":"0.100000","orderStatus":"FUNDED","createdDate":"2023-06-23 19:07:24","createdIpAddress":"","errors":"No Error Message Received","documentKey":"","esignStatus":"NOTSIGNED","users":"","field1":"","field2":"","field3":"","RRApprovalStatus":"Pending","RRName":"","RRApprovalDate":"","PrincipalApprovalStatus":"Pending","PrincipalName":"","PrincipalDate":"","archived_status":"0","closeId":"","eligibleToClose":"no","tradeId":"20XXXXXX"} |
updateTradeTransactionType | This webhook is triggered when the transaction type (ex: Check, ACH, Wire, etc.) of a trade is changed. | {"tradeId":"542XXXXXX","transactionType":"CreditCard"} |
updateNdaDocuSignStatus | This webhook is triggered when the NDA Docusign signature status has been updated. | |
uploadAccountDocument | This webhook is triggered when a document is uploaded and attached to an account. | {"accountId":"A36XXXXXX"} |
uploadPartyDocument | This webhook is triggered when a document is uploaded and attached to a party. | {"partyId":"P58XXXXXX"} |
uploadTradeDocument | This webhook is triggered when a document is uploaded and attached to a trade ID. | |
uploadVerificationDocument | This webhook is triggered when a document is uploaded for accreditation verification. |
Updated 8 months ago